The try statement lets you test a block of code for errors.
The catch statement lets you handle the error.
The throw statement lets you create custom errors.
The finally statement lets you execute code, after try and catch, regardless of the result.
Errors Will Happen!
When executing JavaScript code, different errors can occur.
Errors can be coding errors made by the programmer, errors due to wrong input, and other unforeseeable things:
<!DOCTYPE html>
<html>
<body>
<p id="demo"></p>
<script>
try {
adddlert("Welcome guest!");
}
catch(err) {
document.getElementById("demo").innerHTML = err.message;
}
</script>
</body>
</html>
In the example above we have made a typo in the code (in the try block).
The catch block catches the error, and executes code to handle it.
JavaScript try and catch
The try statement allows you to define a block of code to be tested for errors while it is being executed.
The catch statement allows you to define a block of code to be executed, if an error occurs in the try block.
The JavaScript statements try and catch come in pairs:
try {
Block of code to try
}
catch(err) {
Block of code to handle errors
}
JavaScript Debugging
It is difficult to write JavaScript code without a debugger.
Your code might contain syntax errors, or logical errors, that are difficult to diagnose.
Often, when JavaScript code contains errors, nothing will happen. There are no error messages, and you will get no indications where to search for errors.
Normally, errors will happen, every time you try to write some new JavaScript code.
JavaScript Debuggers
Searching for errors in programming code is called code debugging.
Debugging is not easy. But fortunately, all modern browsers have a built-in debugger.
Built-in debuggers can be turned on and off, forcing errors to be reported to the user.
With a debugger, you can also set breakpoints (places where code execution can be stopped), and examine variables while the code is executing.
Normally, otherwise follow the steps at the bottom of this page, you activate debugging in your browser with the F12 key, and select "Console" in the debugger menu.
The console.log() Method
If your browser supports debugging, you can use console.log() to display JavaScript values in the debugger window:
<!DOCTYPE html>
<html>
<body>
<h1>My First Web Page</h1>
<script>
a = 5;
b = 6;
c = a + b;
console.log(c);
</script>
</body>
</html>
Javascript Error & Troubleshoot
Action to Be Performed
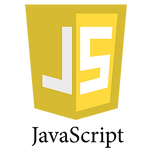