JavaScript strings are used for storing and manipulating text.
A JavaScript string simply stores a series of characters like "John Doe".
A string can be any text inside quotes. You can use single or double quotes:
<!DOCTYPE html>
<html>
<body>
<p id="demo"></p>
<script>
var carName1 = "Volvo XC60";
var carName2 = 'Volvo XC60';
document.getElementById("demo").innerHTML =
carName1 + "<br>" + carName2;
</script>
</body>
</html>
String Length
The length of a string is found in the built in property length:
var txt = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
var sln = txt.length;
Special Characters
Because strings must be written within quotes, JavaScript will misunderstand this string:
var y = "We are the so-called "Vikings" from the north."
The string will be chopped to "We are the so-called ".
The solution to avoid this problem, is to use the \ escape character.
The backslash escape character turns special characters into string characters:
var x = 'It\'s alright';
var y = "We are the so-called \"Vikings\" from the north."
Strings Can be Objects
Normally, JavaScript strings are primitive values, created from literals: var firstName = "John"
But strings can also be defined as objects with the keyword new: var firstName = new String("John")
var x = "John";
var y = new String("John");
// typeof x will return string
// typeof y will return object
Javascript String Object
Performe Text Manipulations
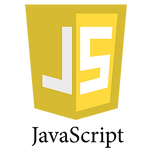