Common HTML Events
Here is a list of some common HTML events:
EventDescription
onchange: An HTML element has been changed
onclick: The user clicks an HTML element
onmouseover: The user moves the mouse over an HTML element
onmouseout: The user moves the mouse away from an HTML element
onkeydown: The user pushes a keyboard key
onload: The browser has finished loading the page
1.ONBLUR:
<!DOCTYPE html>
<html>
<head>
<script>
function myFunction() {
var x = document.getElementById("fname");
x.value = x.value.toUpperCase();
}
</script>
</head>
<body>
Enter your name: <input type="text" id="fname" onblur="myFunction()">
<p>When you leave the input field, a function is triggered which transforms the input text to upper case.</p>
</body>
</html>
2.ONCHANGE:
<!DOCTYPE html><html><head><script>function myFunction() { var x = document.getElementById("fname"); x.value = x.value.toUpperCase();}</script></head><body>Enter your name: <input type="text" id="fname" onchange="myFunction()"><p>When you leave the input field, a function is triggered which transforms the input text to upper case.</p></body></html>
3.ONDBCLICK:
<!DOCTYPE html>
<html>
<head>
<script>
function myFunction() {
document.getElementById("demo").innerHTML = "Hello World";
}
</script>
</head>
<body>
<p ondblclick="myFunction()">Doubleclick this paragraph to trigger a function.</p>
<p id="demo"></p>
</body>
</html>
4.ONCLICK:
<!DOCTYPE html>
<html>
<head>
<script>
function myFunction() {
document.getElementById("demo").innerHTML = "Hello World";
}
</script>
</head>
<body>
<p>Click the button to trigger a function.</p>
<button onclick="myFunction()">Click me</button>
<p id="demo"></p>
</body>
</html>
5.ONFOCUS:
<!DOCTYPE html>
<html>
<head>
<script>
function myFunction(x) {
x.style.background = "yellow";
}
</script>
</head>
<body>
Enter your name: <input type="text" onfocus="myFunction(this)">
<p>When the input field gets focus, a function is triggered which changes the background-color.</p>
</body>
</html>
6.ONKEYDOWN:
<!DOCTYPE html><html><head><script>function myFunction() { alert("You pressed a key inside the input field");}</script></head><body><p>A function is triggered when the user is pressing a key in the input field.</p><input type="text" onkeydown="myFunction()"></body></html>
7.ONKEYUP:
<!DOCTYPE html>
<html>
<head>
<script>
function myFunction() {
var x = document.getElementById("fname");
x.value = x.value.toUpperCase();
}
</script>
</head>
<body>
<p>A function is triggered when the user releases a key in the input field. The function transforms the character to upper case.</p>
Enter your name: <input type="text" id="fname" onkeyup="myFunction()">
</body>
</html>
8.ONKEYPRESS:
<!DOCTYPE html><html><head><script>function myFunction() { alert("You pressed a key inside the input field");}</script></head><body><p>A function is triggered when the user is pressing a key in the input field.</p><input type="text" onkeypress="myFunction()"></body></html>
9.ONLOAD:
<!DOCTYPE html>
<html>
<head>
<script>
function myFunction() {
alert("Page is loaded");
}
</script>
</head>
<body onload="myFunction()">
<h1>Hello World!</h1>
</body>
</html>
10.ONUNLOAD:
<!DOCTYPE html><html><head><script>function myFunction() { alert("Thank you for visiting W3Schools!");}</script></head><body onunload="myFunction()"><h1>Welcome to my Home Page</h1><p>Close this window or press F5 to reload the page.</p></body></html>
Javascript Events
Add Events To Your Page
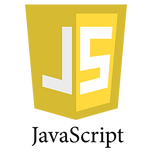